Notes Chapter 4 Conditional and Iterative Statements
Introduction
- Generally a program executes from starting point to end point.
- Some program does not execute in order.
- As per the requirement, execution order of the program can be changed and it is also possible to execute a program repeatedly.
- Python provides control structures to manage the order of execution of a program, which are if-else, for, while and jump statements like break, continue.
Types of statements
In Python, statements are of 3 types-
» Empty Statements
• pass
» Simple Statements (Single Statement)
• name=input (“Enter your Name “)
• print(name) etc.
» Compound Statements

•Here, Header line starts with the keyword and ends at colon (:).
•The body consists of more than one simple Python statements or compound statements.
Statement Flow Control
In a program, statements executes in sequential manner or in selective manner or in iterative manner.

Program Logic Development Tool
A program has following development stages-
- Identification of the problem
- Analysis of problem
- Writing Algorithm or Designing Flowchart
- Writing Code
- Testing and Debugging
- Implementation
- Maintenance
Algorithm
A process or set of rules to be followed in problem-solving operations is an algorithm.
For ex-
Algorithm to add two numbers is as under-
- Input First Number
- Input Second Number
- Add First Number with Second Number and store into Third number.
- Display Third number
Flowcharts
A flowchart is a graphical representation of an algorithm, workflow or process. The flowchart shows the steps as boxes of various kinds, and their order by connecting the boxes with arrows.
For ex- flowchart to calculate simple interest is as under-
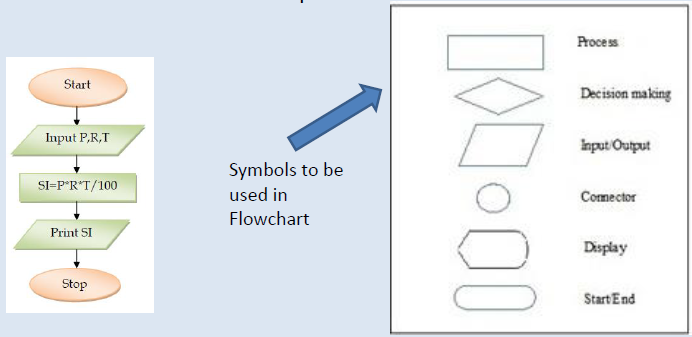
if Statement
In Python, if statement is used to select statement for processing. If execution of a statement is to be done on the basis of a condition, if statement is to be used. Its syntax is-
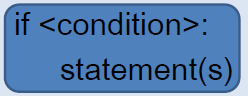
like –
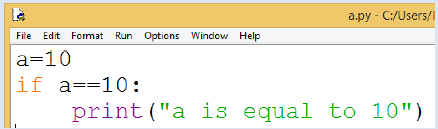
if-else Statement
If out of two statements, it is required to select one statement for processing on the basis of a condition, if-else statement is to be used. Its syntax is-
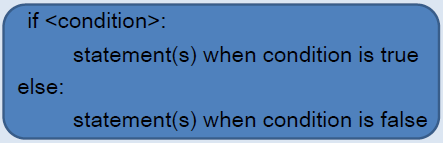
like –
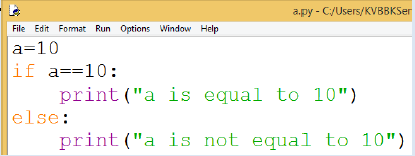
if-elif Statements
If out of multiple statements, it is required to select one statement for processing on the basis of a condition, if-elif statement is to be used. Its syntax is-
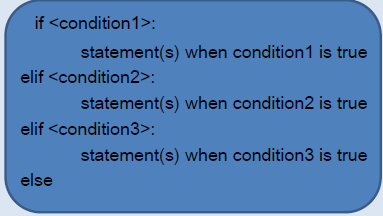
like –
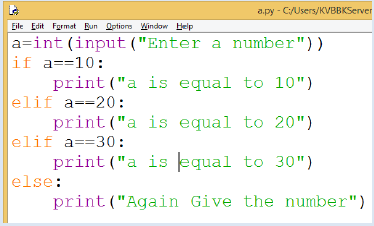
Nested If -else
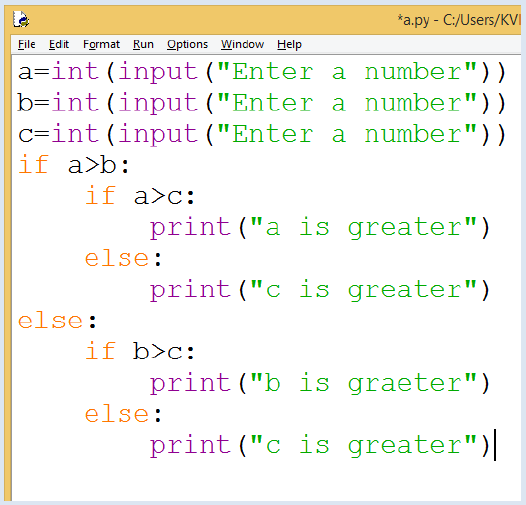
Loop/ Repetition/ Iteration
These control structures are used for repeated execution of statement(s) on the basis of a condition. Loop has 3 main components-
1. Start (initialization of loop)
2. Step (moving forward in loop )
3. Stop (ending of loop)
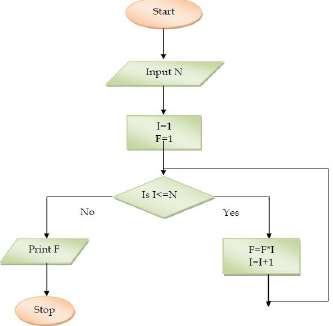
Python has following loops-
–for loop
–while loop
range () Function
In Python, an important function is range( ). its syntax is-
range ( <lower limit>,<upper limit>)
If we write – range (0,5 )
Then a list will be created with the values [0,1,2,3,4] i.e. from lower limit to the value one less than ending limit.
range (0,10,2) will have the list [0,2,4,6,8].
range (5,0,-1) will have the list [5,4,3,2,1].
in and not in operator
in operator-
3 in [1,2,3,4] will return True.
5 in [1,2,3,4] will return False.
not in operator-
5 not in [1,2,3,4] will return True.
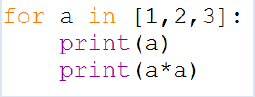
Table of a number by For loop
Syntax of For Loop
for <var> in <sequence>:
<statements to repeat>
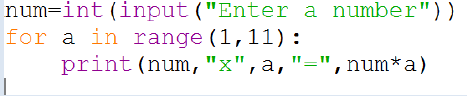
Output
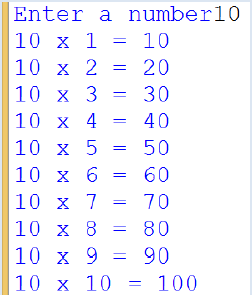
Table of a number by while loop
Syntax of While Loop
While <LogicalExpression>:
<loop body with increment
or decrement>
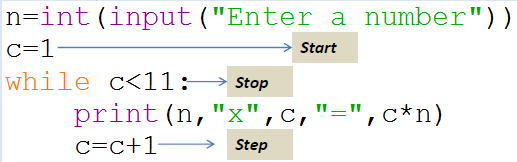
Output
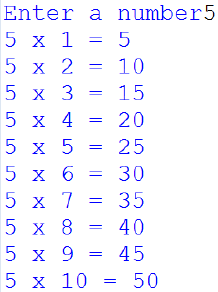
Jump Statements
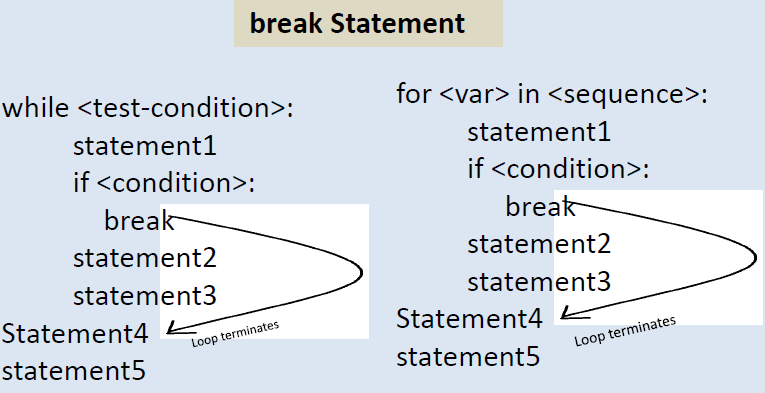
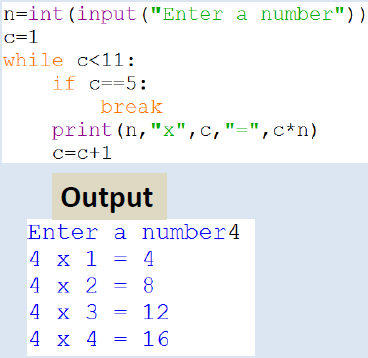
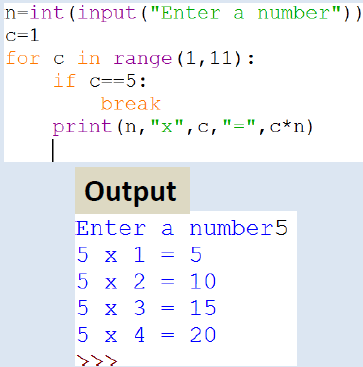


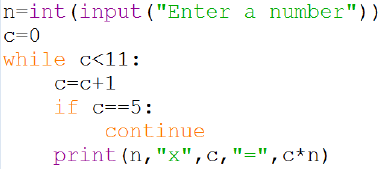
Output of both the programs
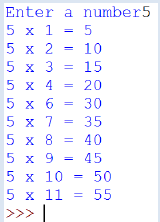
Nested Loop
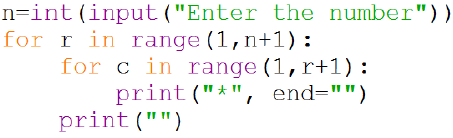
Output
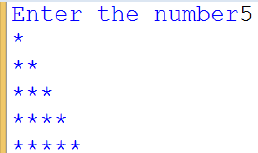
