Notes Chapter 8 Tuple Manipulation
Introduction
• In Python, tuple is also a kind of container which can store list of any kind of values.
• Tuple is an immutable data type which means we can not change any value of tuple.
• Tuple is a sequence like string and list but the difference is that list is mutable whereas string and tuple are immutable.
• In this chapter we will see manipulation on tuple i.e. creation of tuple, its use and operations on tuple with built in functions.
Creation of Tuple
• In Python, “( )” parenthesis are used for tuple creation
( ) empty tuple
( 1, 2, 3) integers tuple
( 1, 2.5, 3.7, 7) numbers tuple
(‘a’, ’b’, ’c’ ) characters tuple
( ‘a’, 1, ‘b’, 3.5, ‘zero’) mixed values tuple
(‘one’, ’two’, ’three’, ’four’) string tuple
*Tuple is an immutable sequence whose values can not be changed.
Look at following examples of tuple creation carefully-
Empty tuple:

Single element tuple:

Long tuple:

Nested tuple:

tuple() function is used to create a tuple from other sequences. See examples-

Accessing a Tuple
• In Python, the process of tuple accessing is same as with list. Like a list, we can access each and every element of a tuple.
• Similarity with List- like list, tuple also has index. All functionality of a list and a tuple is same except mutability.

• len ( ) function is used to get the length of tuple.
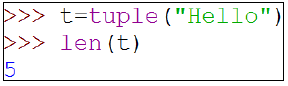
• Indexing and Slicing:
* T[ i ] returns the item present at index i.
* T[ i : j ] returns a new tuple having all the items of T from index i to j.
* T [ i : j : n ] returns a new tuple having difference of n elements of T from index i to j.

• Membership operator:
* Working of membership operator “in” and “not in” is same as in a list. (for details see the chapter- list manipulation).
* Concatenation and Replication operators:
* + operator adds second tuple at the end of first tuple. * operator repeats elements of tuple.
• Accessing Individual elements-

• Traversal of a Tuple –

Tuple Operations
• Tuple joining
* Both the tuples should be there to add with +.
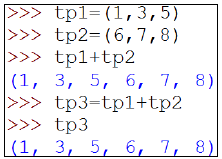
• Some errors in tuple joining-
* In Tuple + number
* In Tuple + complex number
* In Tuple + string
* In Tuple + list
* Tuple + (5) will also generate error because when adding a tuple with a single value, tuple will also be considered as a value and not a tuple.
• Tuple Replication-
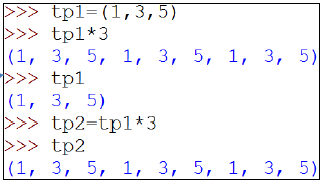
Tuple Slicing

Tuple Comparision
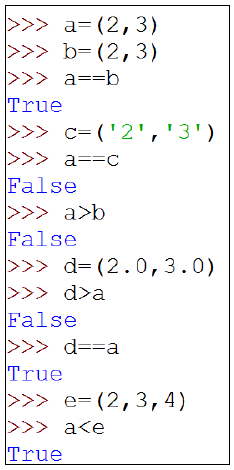
Tuple unpacking

Tuple Deletion
As we know that tuple is of immutable type, it is not possible to delete an individual element of a tuple. With del() function, it is possible to delete a complete tuple. Look at following example-

Tuple Functions

