Sample Paper Class 12 Computer Science Term 1 Set C
Section A
1. In this type conversion, Python automatically converts one data type to another data type.
(a) Explicit
(b) Implicit
(c) Both (a) and (b)
(d) None
Answer
B
2. Which of the symbol is used for processing in flowchart?

Answer
C
3. Which of the following is a valid identifier?
(a) import
(b) Sum^2
(c) Num123
(d) Sum Func
Answer
C
4. Observe the below code and find the output from the following options.
t=“LEARN”
t1=tuple(t)
print(t1)
(a) (L, E, A, R, N)
(b) (‘L’, ‘E’, ‘A’, ‘R’, ‘N’)
(c) [‘L’, ‘E’, ‘A’, ‘R’, ‘N’]
(d) Error
Answer
B
5. Which of the following is also called iterate over a tuple?
(a) Slicing
(b) Accessing
(c) Replicating
(d) Traversing
Answer
D
6. What is the output of following code?
>>>t=(45, 89, 9, ‘The’, 23, 25)
>>>t.index(‘The’)
(a) 3
(b) 4
(c) − 4
(d) −2
Answer
A
7. Which of the following method will force out any unsaved data that exists in a program buffer to the actual file?
(a) dump()
(b) load()
(c) seek()
(d) flush()
Answer
D
8. Which mode is used for appending in binary file?
(a) a+
(b) a
(c) ab+
(d) aw+
Answer
C
9. Ansh intends to position the file pointer to the end of a text file. Identify the Python statement for the same assuming F is the Fileobject.
(a) F.tell(2)
(b) F.seek(0)
(c) F.seek(1)
(d) F.seek(2)
Answer
D
10. File extension to save the CSV file is
(a) .c
(b) .csv
(c) .cv
(d) .cs
Answer
B
12. What is the output of following code?
dic1={“One” : 45, “Two” : 72}
print(list(dic1.keys()))
(a) [“One” : 45, “Two” : 72]
(b) [“One”, “Two”]
(c) (“One”; “Two”)
(d) (“One” : 45, “Two” : 72)
Answer
B
13. What will be the output?
a=int(53.46 + 3/3)
print(a)
(a) 53
(b) 54
(c) 54.46
(d) 53.47
Answer
B
15. Observe the following code :
def Total(a,b):
print (a+b)
x = 2
Total (3, x)
In given code, a, b denotes the
(a) arguments
(b) parameters
(c) functions
(d) values
Answer
B
16. What data type is the object L?
L = [23, ‘Computer’, 12.5, 56, 8]
(a) list
(b) dictionary
(c) array
(d) tuple
Answer
A
17. Which of the following is the truncation division operator?
(a) /
(b)%
(c) //
(d)|
Answer
C
18. What happens, if a local variable exists with the same name as the global variable you want to access?
(a) The local variable is shadowed
(b) Undefined behavior
(c) The global variable is shadowed
(d) Error
Answer
C
19. Which of the following is false about “from-import” form of import?
(a) The syntax is: from modulename import identifier
(b) This form of import prevents name clash
(c) The namespace of imported module becomes part of importing module
(d) The identifiers in module are accessed directly as: identifier
Answer
B
20. To read the remaining lines of the file from a file object infile, we use ………… .
(a) infile.read(n)
(b) infile.read()
(c) infile.readline()
(d) infile.readlines()
Answer
D
21. Which of the following function can accept more than one positional argument?
(a) pickle.dumps()
(b) pickle. loads()
(c) pickle.dump()
(d) pickle. load()
Answer
A
22. Which statement correctly represent the Statement 1 in the given code?
def myFun(val):
______ # Statement 1
print(myFun(82))
(a) True
(b) False
(c) return val
(d) return Flag
Answer
C
23. In file handling, which path does not start with a leading forward slash?
(a) Absolute path
(b) Relative path
(c) Random path
(d) Module path
Answer
B
25. ……… can be defined as an abnormal condition in a program resulting in the disruption to the flow of the program.
(a) Exception
(b) Error
(c) Compile
(d) Debugging
Answer
A
Section B
26. What is the output of following code?
i=1
while (i<10):
if (i= = 5):
break
print(i)
i = i + 1

Answer
A
27. What is the output of following code?
for i in range (− 6, − 10, − 2) :
print (i + 1)
(a) [− 6, − 8]
(b) [− 6, − 4]
(c) [− 4, − 6]
(d) None
Answer
D
28. What is the output of following code?
d={‘P’ : 12, ‘Q’ : 10, ‘R’ : 5, ‘S’ : 13}
if ‘R’ in d :
del d[‘R’]
print(d)
(a) {‘P’ : 12, ‘Q’ : 10, ‘S’ : 13}
(b) [‘P’ : 12, ‘Q’ : 10, ‘S’ : 13]
(c) {‘R’ : 5}
(d) [‘R’ : 5]
Answer
A
29. Observe the code and choose the correct option to fill up the blank marked as Statement 1 to write ‘Learning’.
myfile=open(“Exam.txt”, ‘a’)
______ # Statement 1
myfile.close()
(a) myfile.write()
(b) myfile.write (Learning)
(c) myfile.write (“Learning”)
(d) writelines(“Learning”)
Answer
C
30. What is the output of following code?
i=4
def myFunc():
i=2
global i
i=i+2
print(i)
print(i)
(a) 6
2
(b) 4
2
(c) 6
4
(d) 4
6
Answer
A
31. What will be the output of following code?
import random
arr=[2, 3, 4, 5, 6, 7]
min=random.randint(1, 3)
max=random.randint(2, 4)
for i in range(min, max+1):
print(arr[i], end=“*”)
(a) 1*4*7*
(b) 3*4*5*
(c) 5*6*7*
(d) 4*5*7*
Answer
B
32. What will be the output of the following Python code?
i = 1
while i < 10:
print(i)
i += 3
else:
print(2)

Answer
A
33. What will be the output of the following Python code?
val = (i for i in range(5))
for i in val:
print(i+1)
for i in val:
print(i)
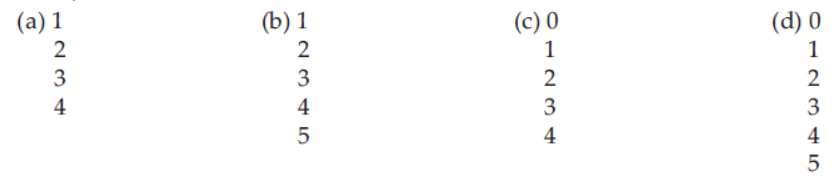
Answer
B
35. Evaluate the following expression and identify the correct answer.
A=10 * 5 + 3 / / 4 + 12 − 8 / /3 + 2 − 1
(a) 61
(b) 62
(c) 63
(d) 64
Answer
A
36. What is the output of following code?
d={ }
a, b, c = 1, 2, 3
d[a, b, c] = a + b − c
a, b, c = 2, 10, 4
d[a, b, c] = a + b − c
print(d)
(a) ((1, 2, 3) : 0, (2, 10, 4) : 8)
(b) [(1, 2, 3) : 0, (2, 10, 4) : 8)]
(c) {(1, 2, 3) : 0, (2, 10, 4) : 8}
(d) Error
Answer
C
37. What is the output of following code?
list1=[ ]
for i in range (10, 30):
if (i%3 = = 0) and (i%2!=0) :
list1.append (str(i))
print(‘,’. join(list1))
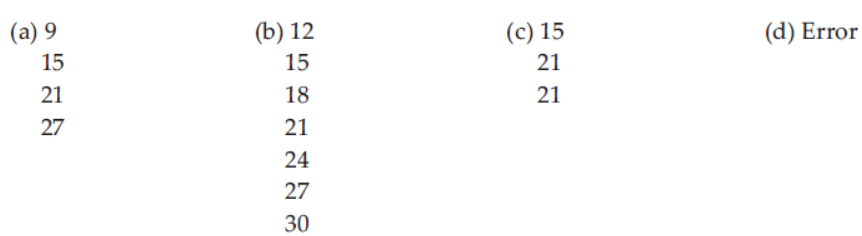
Answer
C
38. What is the output of following code?
d={}
d[1]=5
d[‘1’]=4
d[1.0]=14
add=0
for i in d:
add=add+d[i]
print(add)
(a) 15
(b) 18
(c) 24
(d) 19
Answer
B
39. What is the output of following code?
tup1=(14,)
tup2=()
t=tup1+tup2
any(t)
(a) 0
(b) 1
(c) False
(d) True
Answer
D
40. What is the output of following code?
def Func(*str1):
for i in str1:
print(i)
Func(*[“LEARN”])
(a) L, E, A, R, N
(b) [L, E, A, R, N]
(c) [LEARN]
(d) LEARN
Answer
D
41. What is the output of following code?
def test(Learn, n=1)
print(Learn*n)
test(‘Education’)
test(‘Skills’, 2)
(a) Education
SkillsSkills
(b) Education
Skills
(c) EducationEducation
Skills
(d) EducationEducation
SkillsSkills
Answer
A
42. If list val contains What is the output of following code?
def myFun(val):
for i in range (len(val) − 1, − 1, − 1):
print(val[i]*4)
(a) [3, 4, 7, 9, 2, 4, 5]
(b) [5, 4, 2, 9, 7, 4, 3]
(c) [5, 16, 8, 9, 7, 16, 3]
(d) [20, 16, 8, 36, 28, 16, 12]
Answer
D
43. What is the output of following code?
val=7
def test():
val=2
global val
val=val+3
print(val)
print(val)
(a) 10
2
(b) 7
2
(c) 5
7
(d) 7
5
Answer
A
44. What is the output of following code?
def myFunc():
n=24
def myFunc1():
n=45
print(n)
print(n)
myFunc()
myFunc1()
(a) 24
45
(b) 45
24
(c) 45
45
(d) 24
24
Answer
C
45. When list1 is [2, 4, 5, 15, 12], then what is the output of following code?
def Func(list1) :
for i in range (len(list1)):
if(list1[i]%3!=0):
list1[i]=list1[i]*5
print(list1)
(a) [10,20, 25, 75, 60]
(b) [2, 4, 5, 75, 60]
(c) [10, 20, 25, 15, 12]
(d) Error
Answer
C
46. Suppose the content of file “learn.txt” is Find the output.
f=open(“learn.txt”, ‘r’)
s=f.read(11)
print(s)
f.close()
(a) Education h
(b) Education hu
(c) Education hub
(d) Education
Answer
A
47. Suppose the content of file “exam.txt” is What is the output of following code?
f=open(“exam.txt”, ‘r’)
s=f.read(10)
print(s)
s1=f.read(15)
print(s1)
f.close()
(a) Education
hub is study p
(b) Education
hub is study po
(c) Education hub is study p
(d) Error
Answer
B
48. Suppose the content of file ‘‘learn.txt’’ is What is the output of following code?
myfile=open(“learn.txt”, ‘r’)
s=myfile.read()
l1=len(s)
print(l1)
myfile.close()
(a) 29
(b) 28
(c) 24
(d) 25
Answer
A
49. What is the output of following code?
list1=[2, 3, [3, 5], 4]
list1[2][0]=5.4
print(list1)
(a) [2, 3, [3, 5.4], 4]
(b) [2, 3, [5.4, 5], 4]
(c) [2, 3, [5.4, 3, 5], 4]
(d) Error
Answer
B
Section C
Case Study Based Questions
Below is a program to display all the records in a file along with line/record number from file “status.txt”. Answer the questions that follow to execute the program successfully.
f= open (_____ , “r”) #Line 1
count= 0
rec=“ ”
while _____ : #Line 2
rec = f . _____ #Line 3
if rec = = “ ”:
_____ #Line 4
count = _____ #Line 5
print(count, rec, end = “ ”)
_____ #Line 6
51. Choose the correct option to fill up the blank marked as Line 2.
(a) 0
(b) 1
(c) False
(d) True
Answer
D
52. Which function will be used to read the content of file marked as Line 3?
(a) readline()
(b) readlines()
(c) read()
(d) read(n)
Answer
A
53. Choose the correct option to fill up the blank marked as Line 4.
(a) continue
(b) break
(c) goto
(d) label
Answer
B
55. Identify the missing code in Line 6.
(a) f.close
(b) myfile.close
(c) file.close()
(d) f.close()
Answer
D